10 advanced Turbo CLI techniques to boost your development workflow
Discover powerful Turbo CLI strategies and commands that can reduce build times by up to 80% and streamline your JavaScript monorepo workflow. Learn practical tips for caching, filtering, and optimizing your development pipeline.
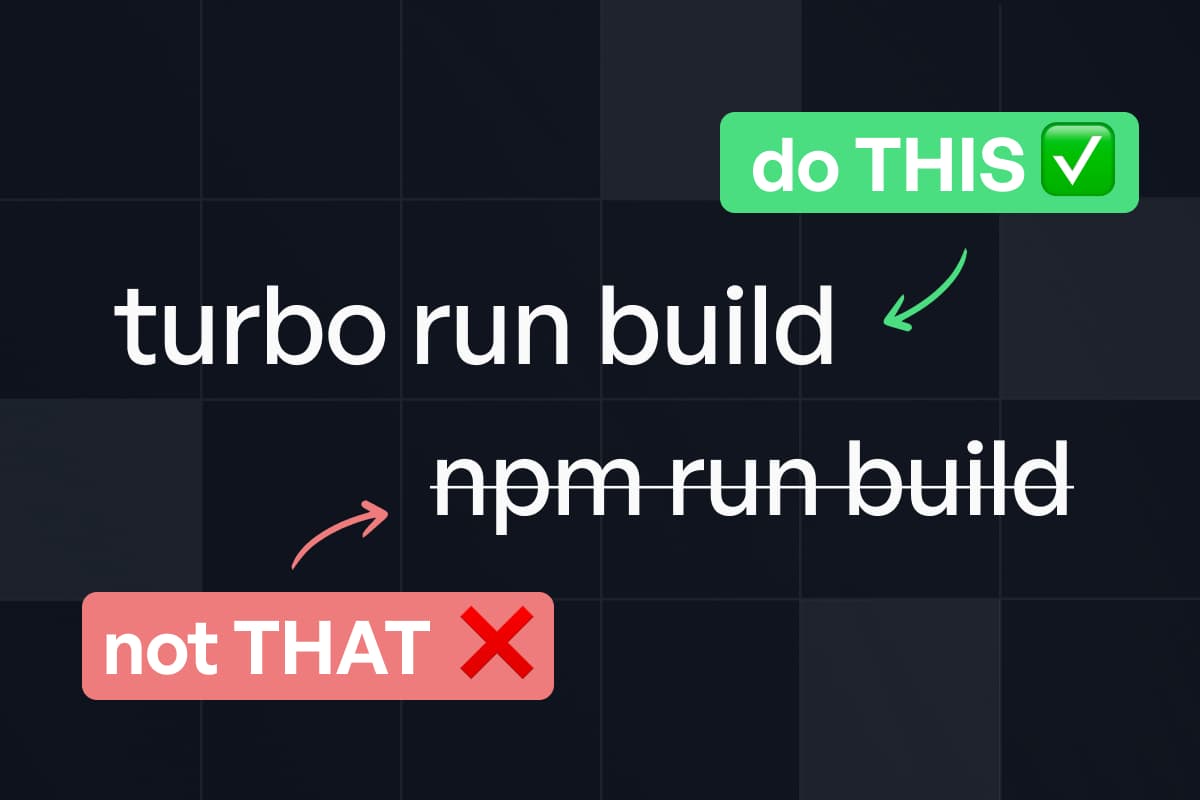
In today's fast-paced JavaScript ecosystem, development velocity can make or break your project's success. With ever-growing codebases and complex dependencies, traditional build tools often struggle to keep up, leading to frustrating wait times and decreased productivity. Turbo CLI has emerged as a game-changing solution to this problem, offering unprecedented build speeds and streamlined workflows for modern development teams.
This guide reveals 10 advanced Turbo CLI techniques that will transform how you manage your JavaScript and TypeScript projects. Whether you're handling a massive monorepo or optimizing a standalone application, these expert strategies will help you achieve dramatic performance improvements and a smoother development experience. If you're new to Turborepo and want to understand the fundamentals first, check out our ultimate guide to Turborepo and modern JavaScript monorepo management.
Why Turbo CLI is revolutionary for JavaScript development
Turbo CLI, the command-line interface for Turborepo, has quickly become an essential tool for JavaScript developers working with complex projects. Built by Vercel and written in Rust for maximum performance, Turbo CLI leverages intelligent caching, parallel execution, and dependency-aware task scheduling to dramatically accelerate build processes.
According to Vercel's benchmarks, teams implementing Turbo CLI have reported build time reductions of 60-85% compared to traditional build systems.
The core innovation of Turbo CLI lies in its approach to task execution. Rather than rebuilding everything on each code change, Turbo intelligently determines what needs to be rebuilt based on your dependency graph and file changes. This targeted approach, combined with sophisticated caching mechanisms, results in lightning-fast incremental builds that can transform your development workflow.
Supercharge your builds with remote caching
One of Turbo CLI's most powerful features is remote caching, which allows you to share build artifacts across your team and CI/CD pipelines. This technique alone can dramatically reduce build times for everyone on your team.
# Log in to enable remote caching
npx turbo login
# Link your project to remote cache
npx turbo link
Once configured, your build artifacts will be automatically uploaded to Vercel's remote cache (or your custom cache server). When another team member needs to build the same code, Turbo will download the cached artifacts instead of rebuilding, saving precious development time.
Remote Caching Benefits
- Reduces CI/CD costs by preventing redundant builds - Ensures consistent build artifacts across environments - Decreases onboarding time for new team members - Accelerates PR verification and deployment processes
For teams with specific security requirements, you can also configure a custom remote cache server:
npx turbo run build --api="https://your-cache-server.com" --token="your-token"
Target exactly what you need with workspace filtering
When working with monorepos containing dozens or hundreds of packages, you rarely need to build everything at once. Turbo CLI's powerful filtering syntax allows you to target specific packages and their dependencies with precision.
# Build only the web app
turbo run build --filter=web
# Build the web app and all its dependencies
turbo run build --filter=web...
# Build all packages that depend on the UI package
turbo run build --filter=...ui
# Build everything except the docs package
turbo run build --filter=!docs
This targeted approach not only speeds up your development cycle but also ensures you're only rebuilding what's necessary. For large monorepos, this technique can reduce build times from minutes to seconds.
New to Turbo CLI or want to skip the configuration hassle?
TurboStarter templates come with pre-configured workspace
filtering and optimized monorepo setups. Get started faster with npx turbostarter new
and focus on building your application, not your tooling.
Make your CI/CD pipelines smarter with change-based filtering
Continuous Integration environments benefit tremendously from Turbo CLI's filtering capabilities. By using Git-based filtering, you can ensure your CI pipeline only builds and tests what's actually changed.
# Only run tasks for packages that changed since the main branch
turbo run build test lint --filter=[main]
# Run for packages changed in the last commit
turbo run build --filter=[HEAD^1]
This technique is particularly valuable for large teams where multiple pull requests might be in progress simultaneously. By focusing CI resources only on what's changed, you can achieve faster feedback cycles and more efficient resource utilization.
When using Git-based filtering in CI environments, ensure your CI system is configured to fetch sufficient Git history for the comparison to work correctly.
Simplify deployments with pruned workspaces
Deploying applications from a monorepo often introduces unnecessary complexity and bloat. Turbo CLI's pruning feature creates a subset of your repository containing only what's needed for a specific application, making deployments faster and more reliable.
# Create a pruned subset for the 'web' application
turbo prune --scope=web
This command generates a new directory (./.turbo/out
) containing:
- The web application and all its dependencies
- A pruned
package.json
with only the necessary dependencies - A correctly configured lockfile
- A minimal
turbo.json
for the pruned workspace
This technique is especially powerful for containerized deployments:
FROM node:18-alpine AS builder
WORKDIR /app
# Copy only package.json files first for better layer caching
COPY .turbo/out/json/ .
COPY .turbo/out/yarn.lock ./
RUN yarn install --frozen-lockfile
# Copy source code
COPY .turbo/out/full/ .
RUN yarn build
Speed up development with persistent tasks
Development servers and watchers are long-running processes that don't fit the typical build-and-exit model. Turbo CLI supports these persistent tasks with dedicated configuration options.
{
"pipeline": {
"dev": {
"cache": false,
"persistent": true,
"dependsOn": ["^build"]
}
}
}
The persistent
flag tells Turbo that this task is long-running and shouldn't block the execution of other tasks. Combined with the cache: false
setting, this ensures your development server always reflects the latest changes.
For even more efficient development workflows, you can run multiple development servers in parallel:
turbo run dev --parallel --filter=web --filter=api
This command starts both your web frontend and API servers simultaneously, with each output clearly labeled for easy identification.
Create smarter build flows with strategic dependencies
One of Turbo CLI's most powerful features is its understanding of task dependencies across packages. By carefully configuring your dependsOn
relationships, you can ensure tasks execute in the optimal order while still maximizing parallelism.
{
"pipeline": {
"build": {
"dependsOn": ["^build"],
"outputs": ["dist/**", ".next/**"]
},
"test": {
"dependsOn": ["build"],
"inputs": ["src/**/*.ts", "test/**/*.ts"]
},
"deploy": {
"dependsOn": ["build", "test", "lint"]
}
}
}
In this configuration:
- A package's
build
task depends on thebuild
task of all its dependencies (^build
) - The
test
task depends on thebuild
task of the same package - The
deploy
task depends onbuild
,test
, andlint
tasks of the same package
This dependency structure ensures everything builds in the correct order while still allowing Turbo to parallelize independent tasks.
Fine-tune caching with smart input and output patterns
Turbo's caching system depends on correctly specifying what files are inputs to and outputs from each task. Fine-tuning these configurations can dramatically improve cache performance.
{
"pipeline": {
"build": {
"inputs": ["src/**", "package.json", "tsconfig.json"],
"outputs": ["dist/**", "!dist/**/*.map"]
}
}
}
By explicitly listing input patterns, you tell Turbo exactly what files should trigger a cache invalidation. Similarly, the output patterns specify what files should be saved in the cache.
Identify your task's actual inputs Not every file in your package affects every task. For test tasks, you might only care about source and test files, not documentation or configuration.
Be specific about outputs Include only the files that are actually produced by the task. Exclude large, non-essential files like source maps when possible.
Use negation patterns Patterns like !dist/**/*.map
exclude files that
match the pattern, allowing for fine-grained control.
Visualize and optimize your build process
To optimize what you can't measure, Turbo CLI provides powerful profiling tools that help visualize your build process and identify bottlenecks.
# Generate a detailed profile of your build
turbo run build --profile=build-profile.json
# Create a dependency graph visualization
turbo run build --graph=build-graph.html
The profile file can be loaded into Chrome's tracing tool (navigate to chrome://tracing
) to provide a detailed timeline view of your build process. This visualization shows exactly how long each task takes and how they relate to each other, making it easy to identify optimization opportunities.
The graph visualization provides a different perspective, showing the dependency relationships between tasks as a directed graph. This can help identify unnecessary dependencies or opportunities for better parallelization.
Control environment variables for reliable caching
Environment variables can significantly impact build outputs, potentially leading to cache invalidation issues. Turbo CLI provides granular control over how environment variables affect caching.
{
"pipeline": {
"build": {
"dependsOn": ["^build"],
"env": ["NODE_ENV", "NEXT_PUBLIC_*", "!SECRET_*"],
"outputs": ["dist/**"]
}
}
}
The env
array specifies which environment variables should be considered when determining whether to use cached results. In this example:
NODE_ENV
- The exact value of this variable affects cachingNEXT_PUBLIC_*
- Any environment variable starting withNEXT_PUBLIC_
affects caching!SECRET_*
- Environment variables starting withSECRET_
are explicitly excluded from cache consideration
This technique ensures your builds use cached results appropriately even when environment variables change.
Set up custom caching for enterprise environments
For enterprise teams with specific security or compliance requirements, Turbo CLI supports custom remote cache servers. This allows you to keep build artifacts within your organization's infrastructure while still benefiting from remote caching.
{
"$schema": "https://turbo.build/schema.json",
"remoteCache": {
"signature": true,
"url": "https://your-internal-cache-server.com"
},
"pipeline": {
// pipeline configuration...
}
}
Implementing a custom remote cache server requires:
- A server that implements Turbo's cache API
- Authentication mechanisms for cache access control
- Storage infrastructure for the cached artifacts
For high-security environments, you can also enable cache signing using the signature
option, which ensures cache artifacts haven't been tampered with.
For AWS environments, you can implement a remote cache using: - S3 for artifact storage - Lambda for the API layer - CloudFront for global distribution - IAM for access control
Azure implementations typically use: - Blob Storage for artifacts - Azure Functions for the API - Azure CDN for distribution - Azure AD for authentication
Self-hosted options include: - A custom server using Express.js or similar - Local or network file storage - Basic authentication mechanisms - Docker containers for easy deployment
Real-world impact: Case studies
The theoretical benefits of Turbo CLI are compelling, but how does it perform in real-world scenarios? Let's examine some concrete examples.
E-Commerce Platform Migration
A large e-commerce company migrated their monorepo from a traditional npm scripts setup to Turbo CLI. The results were dramatic:
- CI build times reduced from 22 minutes to 4 minutes
- Local development builds accelerated from 3 minutes to 15 seconds
- Developer satisfaction scores increased by 35%
- Deployment frequency improved by 60%
The key factors in their success were proper task dependency configuration and effective use of remote caching across their CI/CD pipeline.
Open Source Library Ecosystem
A popular open-source project with 30+ packages implemented Turbo CLI to streamline their development and release process:
- Release process time reduced by 75%
- Contributor onboarding time cut in half
- Testing coverage increased due to faster feedback cycles
- Maintenance burden decreased significantly
By leveraging workspace filtering and pruned subsets, they were able to focus their attention on the specific packages being modified, rather than rebuilding the entire ecosystem for each change.
Transform your development workflow today
Turbo CLI represents a quantum leap forward in build system technology for JavaScript and TypeScript projects. By implementing the ten techniques outlined in this guide, you can dramatically improve your development productivity, reduce infrastructure costs, and deliver software faster than ever before.
The key takeaways include:
- Remote caching can reduce build times by 60-85% across teams
- Strategic filtering ensures you only build what's necessary
- Optimized CI/CD pipelines focus resources where they matter most
- Proper dependency configuration maximizes parallelization opportunities
- Profiling tools help identify and eliminate bottlenecks
Ready to transform your development workflow? Start implementing these Turbo CLI techniques today and experience the difference in your productivity and build times.
Whether you're managing a massive enterprise monorepo or a focused single-package application, Turbo CLI provides the tools you need to build faster, smarter, and more efficiently. The time you save waiting for builds can be redirected to what truly matters: creating exceptional software that delivers value to your users.
For an even faster start, check out our TurboStarter templates with pre-configured Turbo CLI setups for web, mobile, and browser extension projects. Or run our streamlined CLI with npx turbostarter new
to get a production-ready project in seconds. If you want to learn more about the TurboStarter CLI, read our guide on the only turbo CLI you need to start your next project in seconds.
✔ All prerequisites satisfied.ℹ Configuring web app...✔ API installed.✔ Billing setup created.✔ Emails configuration done.ℹ Configuring mobile app...✔ Authentication set up.✔ Published to stores.ℹ Configuring browser extension...✔ Themes installed.✔ Connected with web app.✔ Project initialization completed.
Happy building! 🚀